mininet 實戰
Topo
: Mininet拓撲結構的base class
build()
: The method to override in your topology class. Constructor parameters (n) will be passed through to it automatically by Topo.init().
addSwitch()
: 給拓撲結構中增加一個switch,返回switch名字
addHost()
: 增加host,返回host名字
addLink()
: 增加一個雙向link,返回link的key
Mininet
: 建立和管理網路的main class
start()
: 開啟網路
pingAll()
: 通過host之間的互ping測試連通性
stop()
: 關閉網絡
net.hosts
: 網路中的所有主機
dumpNodeConnections()
: 所有的連接關係
setLogLevel( 'info' | 'debug' | 'output' )
: set Mininet's default output level; 'info' is recommended as it provides useful information.
5.1. 目標
- 啟動50個
hosts
,作為VM server farm - 啟動1個
controller
- 啟動1個
switch
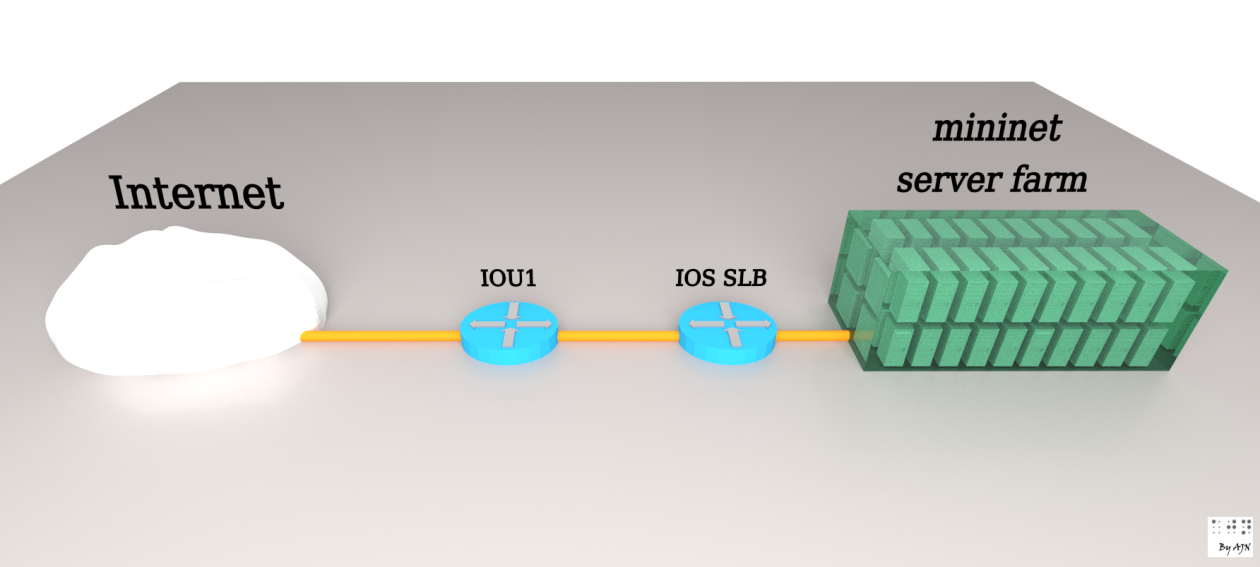
5.2. Code
#!/usr/bin/python
import re
from mininet.net import Mininet
from mininet.node import Controller
from mininet.cli import CLI
from mininet.link import Intf
from mininet.log import setLogLevel, info, error
from mininet.util import quietRun
from mininet.node import OVSController
def checkIntf( intf ):
"Make sure intf exists and is not configured."
if ( ' %s:' % intf ) not in quietRun( 'ip link show' ):
error( 'Error:', intf, 'does not exist!\n' )
exit( 1 )
ips = re.findall( r'\d+\.\d+\.\d+\.\d+', quietRun( 'ifconfig ' + intf ) )
if ips:
error( 'Error:', intf, 'has an IP address and is probably in use!\n' )
exit( 1 )
def myNetwork():
net = Mininet( topo=None, build=False)
info( '*** Adding controller\n' )
net.addController(name='c0',controller = OVSController)
info( '*** Add switches\n')
s1 = net.addSwitch('s1')
max_hosts = 50
newIntf = 'enp3s0'
host_list = {}
info( '*** Add hosts\n')
for i in xrange(1,max_hosts+1):
host_list[i] = net.addHost('h'+str(i))
info( '*** Add links between ',host_list[i],' and s1 \r')
net.addLink(host_list[i], s1)
info( '*** Checking the interface ', newIntf, '\n' )
checkIntf( newIntf )
switch = net.switches[ 0 ]
info( '*** Adding', newIntf, 'to switch', switch.name, '\n' )
brintf = Intf( newIntf, node=switch )
info( '*** Starting network\n')
net.start()
CLI(net)
net.stop()
if __name__ == '__main__':
setLogLevel( 'info' )
myNetwork()
5.3. 執行
sudo python example.py
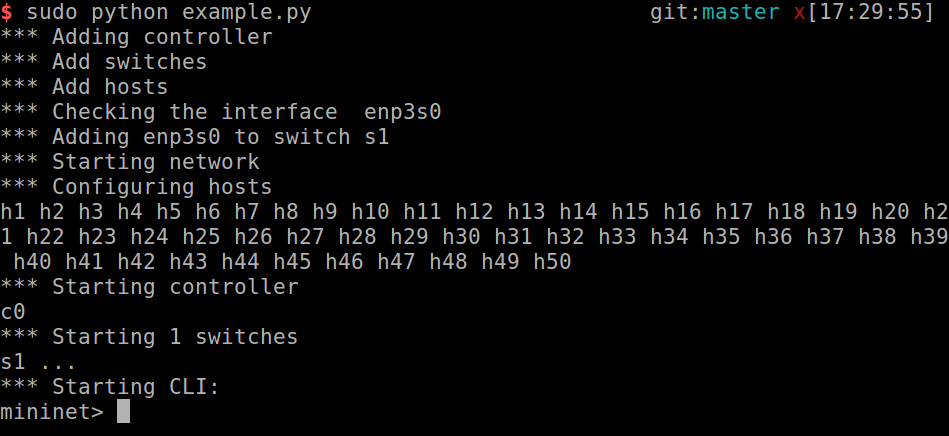
5.4. Q&A
執行出現Cannot find required executable ovs-controller
- 你沒有安裝ovs-controller
sudo apt-get install openvswitch-testcontroller
- ovs-controller是舊名稱,所以你需要複製一下
sudo cp /usr/bin/ovs-testcontroller /usr/bin/ovs-controller
執行出現
Exception: Please shut down the controller which is running on port 6653:
Active Internet connections (servers and established)
tcp 0 0 0.0.0.0:6653 0.0.0.0:* LISTEN 30215/ovs-testcontr
- 你需要kill正在背景執行的ovs-testcontroller
sudo netstat -lptu
sudo service ovs-testcontroller stop
6.1. 底層API
Node
&Link
h1 = Host( 'h1' )
h2 = Host( 'h2' )
s1 = OVSSwitch( 's1', inNamespace=False )
c0 = Controller( 'c0', inNamespace=False )
Link( h1, s1 )
Link( h2, s1 )
h1.setIP( '10.1/8' )
h2.setIP( '10.2/8' )
c0.start()
s1.start( [ c0 ] )
print h1.cmd( 'ping -c1', h2.IP() )
s1.stop()
c0.stop()
6.2. 中層API
net
Object
net = Mininet()
h1 = net.addHost( 'h1' )
h2 = net.addHost( 'h2' )
s1 = net.addSwitch( 's1' )
c0 = net.addController( 'c0' )
net.addLink( h1, s1 )
net.addLink( h2, s1 )
net.start()
print h1.cmd( 'ping -c1', h2.IP() )
CLI( net )
net.stop()
6.3. 上層API
Topology
Object
class SingleSwitchTopo( Topo ):
"Single Switch Topology"
def __init__( self, count=1, **params ):
Topo.__init__( self, **params )
hosts = [ self.addHost( 'h%d' % i ) for i in range( 1, count + 1 ) ]
s1 = self.addSwitch( 's1' )
for h in hosts:
self.addLink( h, s1 )
net = Mininet( topo=SingleSwitchTopo( 3 ) )
net.start()
CLI( net )
net.stop()